Introduction:
A Random Number Generator (RNG) is an essential tool used in various applications, from gaming and simulations to cryptography and statistical analysis. In this tutorial, we will walk you through creating your very own RNG. Whether you’re a beginner or someone with some programming experience, this guide will provide a hands-on experience, helping you understand how RNGs work and how to build one. By the end, you’ll have your own working random number generator ready to use in any project.
Table of Contents:
What is a Random Number Generator?
Importance of RNGs in Modern Applications
Tools Required for the Project
Step-by-Step Guide to Building Your RNG
Step 1: Set Up Your Environment
Step 2: Write the Code for RNG
Step 3: Testing the RNG
Optimizing Your Random Number Generator
Common Issues and How to Solve Them
Conclusion
Content:
1- What is a Random Number Generator?
A Random Number Generator is an algorithm or device designed to produce a sequence of numbers that appear to be random. Unlike deterministic algorithms, RNGs aim to generate numbers without any predictable pattern. RNGs are widely used in cryptography, statistical simulations, gaming, and other fields where unpredictability is required.
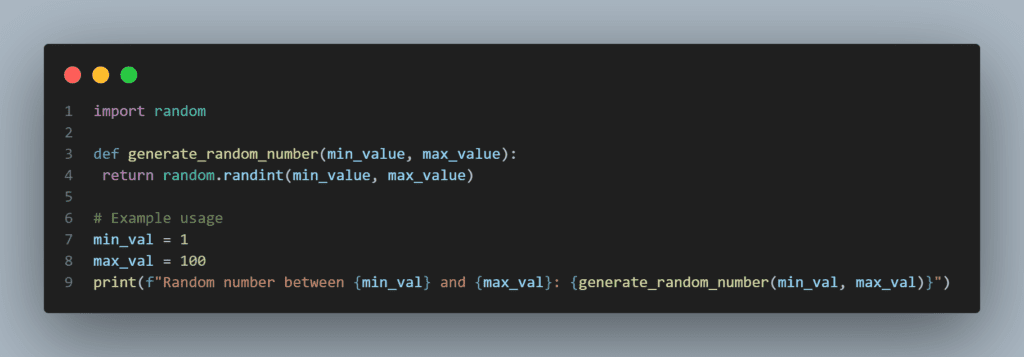

2- Importance of RNGs in Modern Applications
In today’s digital world, RNGs play a crucial role in various sectors:
Gaming: RNGs ensure fairness in games like slot machines, poker, and online roulette.
Cryptography: RNGs provide unpredictable keys for secure data encryption.
Simulations: RNGs are used to model real-world scenarios in areas like weather forecasting, financial modeling, and scientific research. Understanding how to build your own RNG is valuable in learning how these systems work.
3- Tools Required for the Project Before starting, you’ll need the following tools:
A Computer: Any system capable of running programming languages.
Programming Language: We’ll use Python in this tutorial, as it’s beginner-friendly and widely used.
IDE or Text Editor: Tools like Visual Studio Code or PyCharm are perfect for coding.
Libraries: Python’s built-in libraries, like random, will simplify the process.
4- Step-by-Step Guide to Building Your RNG
Step 1: Set Up Your Environment First, make sure Python is installed on your computer. You can download it from the official Python website. Install any necessary IDE or text editor to write your code.
Step 2: Write the Code for RNG Here’s a simple Python code snippet to generate random numbers:
import random
def generate_random_number(min_value, max_value):
return random.randint(min_value, max_value)
# Example usage
min_val = 1
max_val = 100
print(f"Random number between {min_val} and {max_val}: {generate_random_number(min_val, max_val)}")
Example usage
In this code:
We use Python’s random.randint() function to generate a random number between the provided min_value and max_value.
Feel free to modify the min_value and max_value for different outputs.
Step 3: Testing the RNG Now, let’s test your generator. Run the script multiple times and observe the variation in the random numbers. This will help ensure that the RNG is working properly.
5- Optimizing Your Random Number Generator
While the built-in random number generators are sufficient for most uses, sometimes you might want to improve the quality of randomness:
Use Cryptographically Secure RNG: For secure applications like encryption, you can use libraries such as secrets in Python that provide more robust randomness.
Seed Control: Use the random.seed() function to initialize the RNG with a specific seed value to make your results reproducible.
6- Common Issues and How to Solve Them
Non-Randomness: If you notice a pattern or repetition in the output, consider changing the way you seed the RNG or increase the range of numbers.
Performance: If performance becomes an issue with large datasets, consider optimizing the code or using faster libraries like numpy.
7- Conclusion
Building your own Random Number Generator is a fun and educational experience. With just a few lines of code, you can create a basic RNG, and with some optimization, you can enhance its performance for more critical applications. Whether for game development, statistical simulations, or cryptography, RNGs are a powerful tool that you can now integrate into your projects.