Table of Contents
- Introduction
- What You Will Learn
- Tools Needed to Execute the Project
- Skills Required for Project Execution
- Step-by-Step Guide to Building the Game
- Real-World Applications of the Project
- Conclusion
- FAQs
- Tag List
- Meta Description
Introduction
Tic Tac Toe is a timeless two-player game that challenges participants to place X’s and O’s on a 3×3 grid. The goal is to achieve three of the same symbols in a row, column, or diagonal. This project is an excellent way to learn fundamental programming concepts such as array manipulation, conditional logic, and input validation. By building a Tic Tac Toe game, you’ll gain hands-on experience in structuring game logic, debugging, and organizing code efficiently.
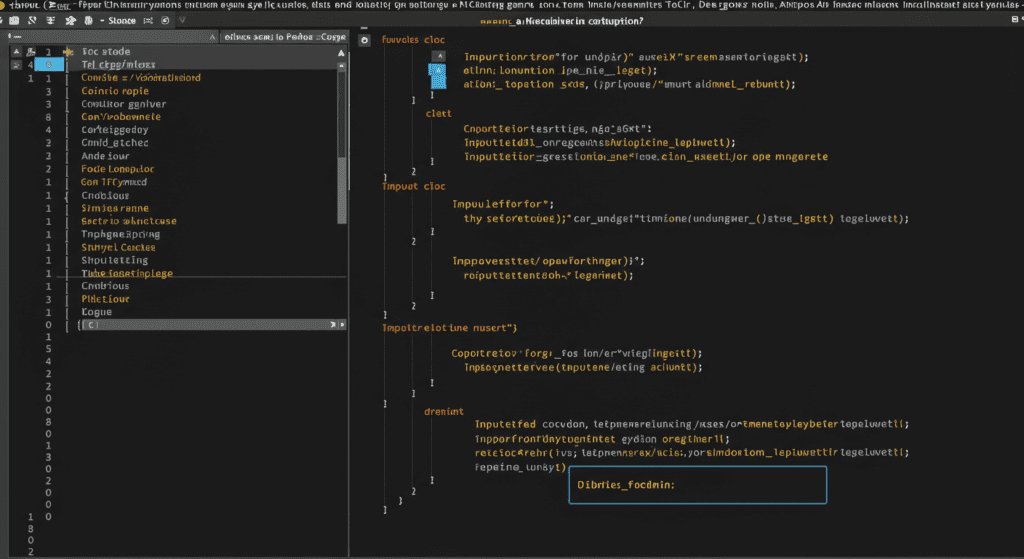
What You Will Learn
Game Logic
You’ll design the core mechanics of the game, including turn-taking, identifying winning combinations, and detecting draws. This involves creating a system that alternates between Player 1 (X) and Player 2 (O) and checks the board’s state after each move.
2D Array Usage
The game board will be represented using a 3×3 array or matrix. You’ll learn how to store and manipulate this data structure to reflect the current state of the game.
Input Validation
Handling invalid moves is a critical aspect of the project. You’ll ensure that players cannot overwrite existing moves or place symbols outside the grid.
Code Organization
Breaking the program into smaller functions will improve readability and maintainability. For example, separate functions can handle board updates, win detection, and user prompts.
Optional AI
For an added challenge, you can implement a basic computer opponent, transforming the game into a single-player experience.
Tools Needed to Execute the Project
Tool | Why Is It Needed? |
---|---|
C Compiler (e.g., GCC, Clang) | Compiles the code and checks for syntax or logic errors. |
Text Editor or IDE (e.g., Code::Blocks) | Provides a workspace for writing, running, and debugging your program. |
Skills Required for Project Execution
- Array Management: Ability to store and manipulate data in a 3×3 grid.
- Conditional Statements: Knowledge of if-else logic to check for wins or draws.
- Loops: Experience with loops to iterate through the grid and manage turns.
- Debugging Techniques: Basic skills to identify and fix errors in the code.
Step-by-Step Guide to Building the Game
- Set Up the Game Board: Create a 3×3 array to represent the grid.
- Player Input: Prompt players to enter their moves (row and column) alternately.
- Update the Board: Place X or O in the selected position and display the updated grid.
- Check for Win or Draw: After each move, call a function to check if a player has won or if the game is a draw.
- Display Results: Announce the winner or declare a draw when the game ends.
- Test Thoroughly: Run multiple tests to ensure the game behaves correctly under various scenarios.
Real-World Applications of the Project
Application | Description |
---|---|
Algorithm Demonstrations | Showcases how simple logic structures operate in real-time. |
Classroom Exercises | Serves as an ideal project for students learning array manipulation and game design. |
Mini-Game Integrations | Acts as a foundation for embedding Tic Tac Toe in larger interactive applications. |
Conclusion
Building a Tic Tac Toe game is an excellent way to practice essential programming skills. From managing arrays to implementing game logic and validating user input, this project covers a wide range of concepts. Whether you’re a beginner or an experienced programmer, this exercise will enhance your understanding of code organization and debugging. Additionally, you can expand the game by adding features like a scoring system or an AI opponent.
FAQs
1. Can I use a single array instead of a 2D array for the game board?
Yes, you can map a single array to a 3×3 grid using indices. For example, index 0-2 can represent the first row, 3-5 the second row, and 6-8 the third row.
2. How do I check for a winning combination?
You can use conditional statements to check all rows, columns, and diagonals for three matching symbols.
3. Can I make the game more visually appealing?
Yes, you can enhance the visuals by using ASCII art or integrating graphical libraries if you’re working in a language that supports them.
4. How do I handle invalid moves?
Use input validation to ensure the selected position is within the grid and not already occupied.
5. Is it possible to create a single-player version?
Yes, you can implement a basic AI that makes random moves or follows simple rules to act as the opponent.