Table of Contents
- Introduction
- What You’ll Need to Build a Virtual Assistant
- Step 1: Define Your Virtual Assistant’s Purpose
- Step 2: Choose the Right Programming Language
- Step 3: Set Up Speech Recognition
- Step 4: Implement Text-to-Speech Functionality
- Step 5: Add Basic Commands and Responses
- Step 6: Integrate APIs for Advanced Features
- Step 7: Test and Improve Your Virtual Assistant
- Conclusion
- FAQs
Introduction
Building a simple virtual assistant may seem complex, but with the right approach, anyone can create a functional AI helper. Whether for automating tasks, answering questions, or controlling smart devices, a DIY virtual assistant can be both educational and practical. This guide walks you through the process step by step, ensuring you understand each stage while keeping the project beginner-friendly.
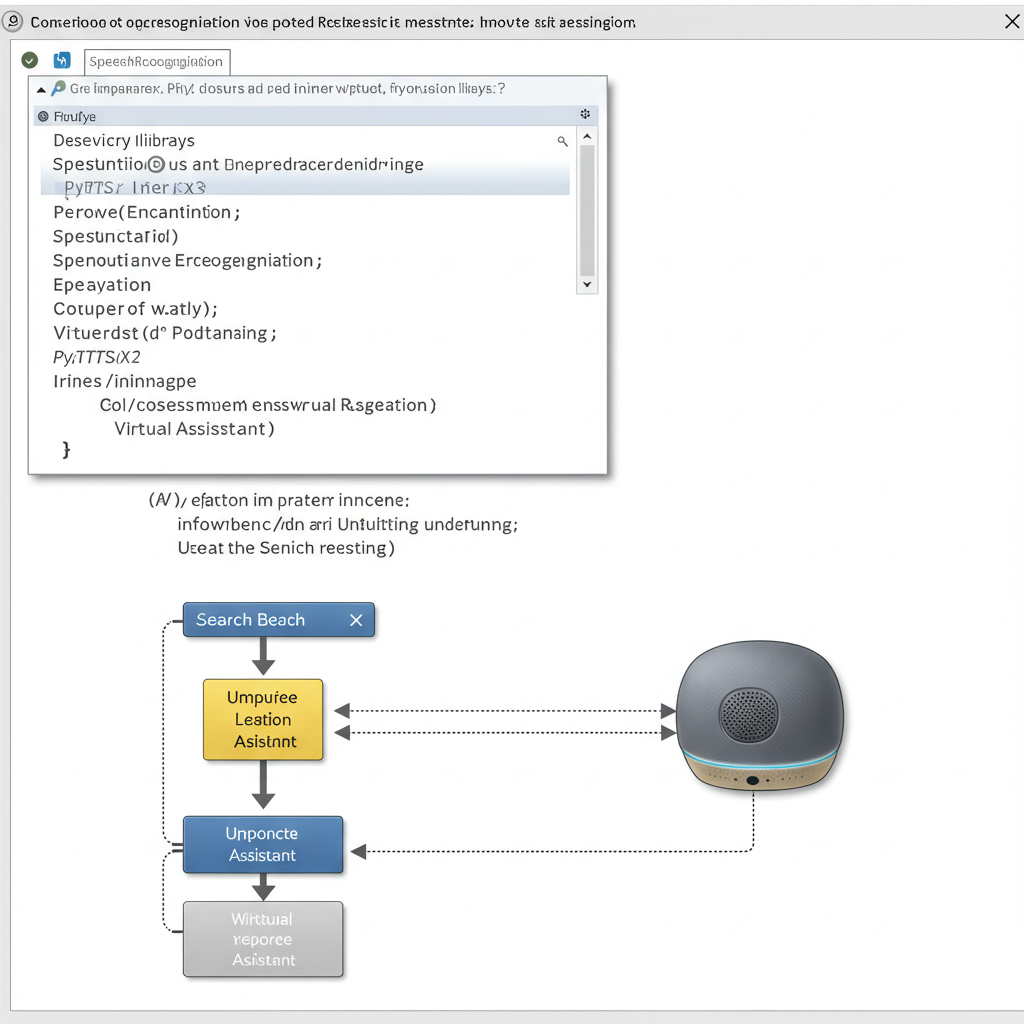
What You’ll Need to Build a Virtual Assistant
Before starting, gather these essentials:
- A computer (Windows, macOS, or Linux)
- Python (recommended for beginners) or another programming language
- Text editor (VS Code, PyCharm, or Sublime Text)
- Internet connection (for API integrations)
- Basic programming knowledge (helpful but not mandatory)
Step 1: Define Your Virtual Assistant’s Purpose
Decide what tasks your virtual assistant should perform:
- Basic voice commands (e.g., “What’s the time?”)
- Web searches & information retrieval
- Smart home control (via IoT integrations)
- Reminders and scheduling
A clear purpose helps streamline development.
Step 2: Choose the Right Programming Language
Python is the best choice for beginners due to its simplicity and robust libraries. Alternatives include:
- JavaScript (Node.js) – For web-based assistants
- Java – For cross-platform compatibility
- C# – For Windows-centric assistants
For this guide, we’ll use Python.
Step 3: Set Up Speech Recognition
Install the speech_recognition
library:
bash
Copy
pip install SpeechRecognition
Basic code to capture voice input:
python
Copy
import speech_recognition as sr recognizer = sr.Recognizer() with sr.Microphone() as source: print("Listening...") audio = recognizer.listen(source) try: text = recognizer.recognize_google(audio) print(f"You said: {text}") except Exception as e: print("Error:", e)
Step 4: Implement Text-to-Speech Functionality
Use pyttsx3
for voice responses:
bash
Copy
pip install pyttsx3
Example code:
python
Copy
import pyttsx3 engine = pyttsx3.init() engine.say("Hello, how can I help you?") engine.runAndWait()
Step 5: Add Basic Commands and Responses
Create a simple command-response system:
python
Copy
def handle_command(text): if "hello" in text.lower(): return "Hi there!" elif "time" in text.lower(): import datetime return f"The time is {datetime.datetime.now().strftime('%H:%M')}" else: return "Sorry, I didn’t understand that." # Integrate with speech recognition user_input = "What's the time?" response = handle_command(user_input) print(response)
Step 6: Integrate APIs for Advanced Features
Enhance functionality with APIs:
- OpenWeatherMap – For weather updates
- Google Custom Search – For web queries
- WolframAlpha – For computational answers
Example (Weather API):
python
Copy
import requests def get_weather(city): api_key = "YOUR_API_KEY" url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}" response = requests.get(url).json() return response['weather'][0]['description'] print(get_weather("London"))
Step 7: Test and Improve Your Virtual Assistant
- Test voice recognition accuracy.
- Expand command responses.
- Optimize performance by reducing latency.
- Add error handling for better reliability.
Conclusion
Building a simple virtual assistant is an exciting project that enhances your programming skills while creating a useful tool. By following these steps, you can develop a basic AI assistant and expand its features over time. Experiment with different APIs and functionalities to make it truly personalized.
FAQs
1. Can I build a virtual assistant without coding?
Yes, using platforms like Dialogflow or Microsoft Bot Framework, but customizability is limited compared to coding your own.
2. Which is the easiest programming language for a virtual assistant?
Python, due to its readability and extensive AI/ML libraries.
3. How do I make my virtual assistant more accurate?
- Use high-quality microphone input.
- Train the model with more voice samples.
- Implement NLP libraries like NLTK or spaCy.
4. Can I run my virtual assistant on a smartphone?
Yes, by converting the script into an app using Kivy (Python) or integrating with mobile APIs.
5. Is an internet connection mandatory?
For speech recognition and API-based features, yes. Offline alternatives like PocketSphinx exist but are less accurate.