Introduction
Are you passionate about coding and looking for a fun way to enhance your JavaScript skills? Developing a small game can be an exciting project that combines creativity and problem-solving, while helping you deepen your understanding of web development. In this DIY tutorial, we will guide you step by step through creating a simple yet engaging JavaScript game that you can easily build on your own. Whether you’re a beginner or have some experience with coding, this tutorial will provide you with the tools you need to develop a game from scratch.
By the end of this tutorial, you will have a fully functional game and a deeper understanding of JavaScript concepts, HTML5, and CSS. Plus, you’ll have a new project to showcase in your portfolio!
Let’s dive into this beginner-friendly JavaScript game development tutorial!
Table of Contents
- Setting Up Your Development Environment
- Requirements
- Tools You Will Need
- How to Get Started
- Understanding the Game Concept
- Defining Your Game Rules
- Deciding the Type of Game (e.g., Puzzle, Platformer, Shooter)
- Creating the Game’s HTML Structure
- Setting Up the HTML File
- Adding Basic Elements to the Page
- Styling the Game with CSS
- Layout Design
- Customizing Game Elements with CSS
- Building the Game’s JavaScript Logic
- Implementing the Game’s Core Mechanics
- Handling User Input
- Managing Game States
- Testing and Debugging Your Game
- Testing for Common Issues
- Debugging with Developer Tools
- Optimizing the Game for Performance
- Best Practices for JavaScript Game Development
- Improving Load Times and Responsiveness
- Publishing Your Game
- Deploying Your Game Online
- Sharing Your Game with the World
1- Setting Up Your Development Environment
Requirements:
Before you start coding, ensure you have the following setup:
A text editor (e.g., VS Code, Sublime Text, or Atom)
A web browser (Google Chrome, Firefox, etc.)
Basic knowledge of HTML, CSS, and JavaScript
Tools You Will Need
JavaScript Frameworks (optional for advanced features)
Web Browser Developer Tools for debugging
How to Get Started
Simply create a new folder for your game project. Inside this folder, create three files:
index.html – For your HTML structure
style.css – For your game’s styles
script.js – For your game’s logic
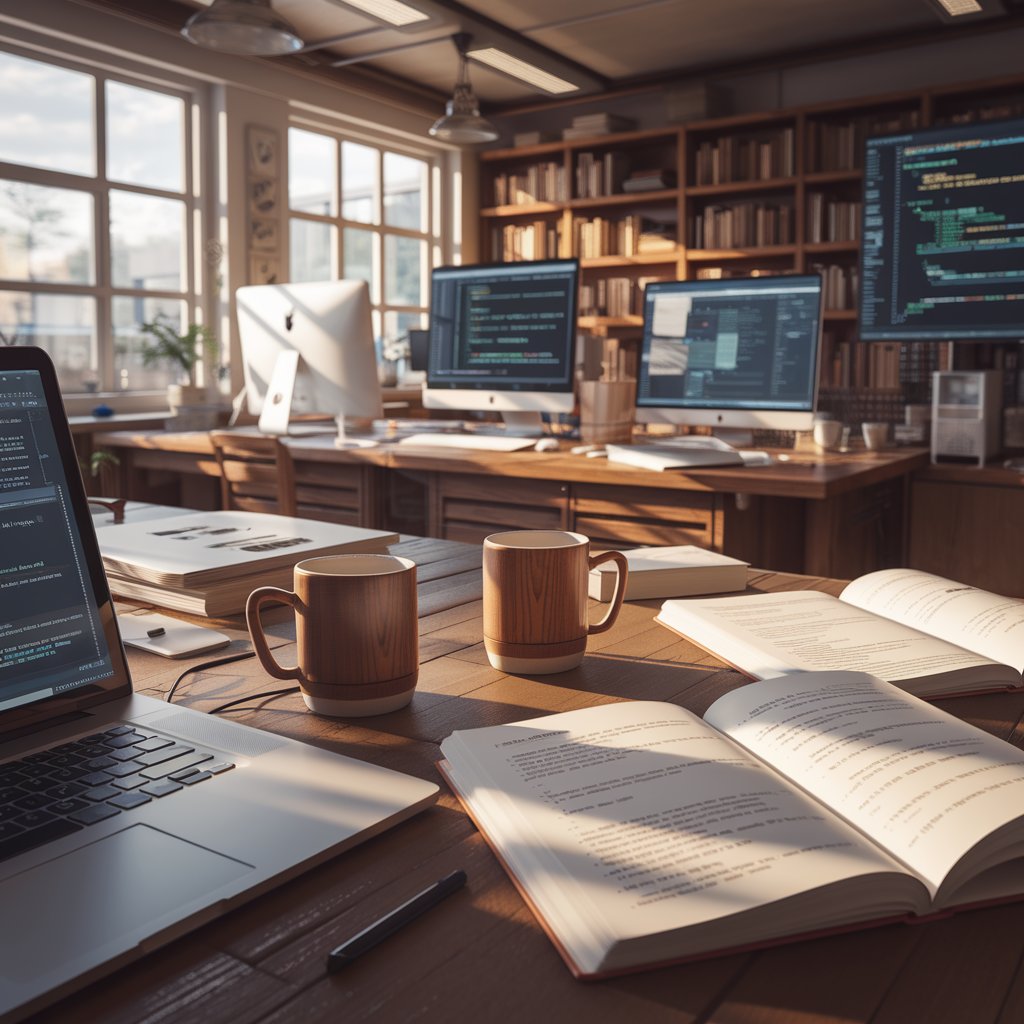
2- Understanding the Game Concept
Defining Your Game Rules
The first step in game development is to decide what type of game you want to create. A simple idea could be a “catch the falling objects” game or a basic click-based game where you need to score points. Here are some basic questions to help you get started:
What is the objective of your game?
How will the user interact with the game?
What are the win and lose conditions?
Deciding the Type of Game
For beginners, simple games like a Tic-Tac-Toe, Memory Game, or Flappy Bird clone are great projects to start with. They are easy to build but still cover the essential concepts of game development.
3- Creating the Game’s HTML Structure
Setting Up the HTML File
The HTML will serve as the foundation for your game. It will define the structure of your webpage, including game elements like buttons, scoreboards, and playable areas.
Here’s a basic structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple JavaScript Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameArea">
<h1>Catch the Falling Objects!</h1>
<div id="gameCanvas"></div>
<button id="startButton">Start Game</button>
<p id="score">Score: 0</p>
</div>
<script src="script.js"></script>
</body>
</html>
Adding Basic Elements to the Page
In the HTML file above, we have a gameArea div that will contain the game elements, such as a title, score display, and a start button. The gameCanvas is where the game action will take place.
4- Styling the Game with CSS
Layout Design
Your game’s style is important to make it visually appealing. Here’s a simple CSS example to style the elements:
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
text-align: center;
margin: 0;
}
gameArea {
padding: 20px;
background-color: #fff;
border-radius: 8px;
max-width: 600px;
margin: 20px auto;
}
gameCanvas {
width: 100%;
height: 300px;
background-color: #eee;
position: relative;
}
button {
padding: 10px 20px;
font-size: 16px;
background-color: #4CAF50;
color: white;
border: none;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
Customizing Game Elements with CSS
You can further customize the appearance of game elements like the falling objects, score display, and buttons. Experiment with different color schemes and animations to make your game more dynamic.
5- Building the Game’s JavaScript Logic
Implementing the Game’s Core Mechanics
In script.js, you’ll define the core logic of your game. For instance, if you’re building a falling objects game, you’ll need to create a function to animate the objects falling down the screen.
let score = 0;
let gameInterval;
function startGame() {
document.getElementById('startButton').disabled = true;
score = 0;
document.getElementById('score').textContent = Score: ${score};
gameInterval = setInterval(gameLoop, 1000 / 60); // Run at 60 FPS
}
function gameLoop() {
// Game logic here (e.g., moving objects, checking collisions)
}
document.getElementById('startButton').addEventListener('click', startGame);
Handling User Input
You'll need to handle user input, such as keypresses or clicks, to interact with the game. For example, if your game involves moving a character, you could use keydown events to capture the player’s movements.
javascript
Copy code
document.addEventListener('keydown', function(event) {
if (event.key === 'ArrowLeft') {
// Move player to the left
} else if (event.key === 'ArrowRight') {
// Move player to the right
}
});
Managing Game States
You’ll need to manage different game states like start, playing, and game over. This helps control the flow of the game.
6- Testing and Debugging Your Game
Testing for Common Issues
Test your game in different browsers to ensure compatibility. Use browser developer tools to debug JavaScript issues, such as console errors or performance lags.
Debugging with Developer Tools
Press F12 in your browser to open Developer Tools. The Console tab will show any JavaScript errors, while the Network tab will help you track resources loading correctly.
7- Optimizing the Game for Performance
Best Practices for JavaScript Game Development
Minimize the use of global variables
Use requestAnimationFrame for smoother animations
Load assets like images asynchronously
Improving Load Times and Responsiveness
Use minification tools to compress your JavaScript and CSS files. Also, consider using lazy loading for large assets.
8- Publishing Your Game
Deploying Your Game Online
You can host your game on a platform like GitHub Pages, Netlify, or Vercel. These platforms offer free hosting for static sites, including HTML, CSS, and JavaScript files.
Sharing Your Game with the World
Once your game is live, share it on social media, forums, and coding communities like Reddit, Dev.to, or CodePen to get feedback and attract players!
Conclusion
Congratulations! You’ve successfully built your first JavaScript game. This tutorial has covered everything from setting up your environment to publishing your game online. Developing games can be a highly rewarding experience, and now you have a working example to add to your portfolio. Keep experimenting and learning new techniques to improve your game development skills!
Happy coding, and best of luck with your next project!