Table of Contents
- Introduction
- What You Will Learn
- Tools Needed to Execute the Project
- Skills Required for Project Execution
- Step-by-Step Guide to Building the Quiz Game
- Real-World Applications of the Project
- Conclusion
- FAQs
Introduction
Creating a Quiz Game in C is an excellent way to enhance your programming skills while building a fun and interactive application. This project tests your ability to structure questions, handle user input, and manage scores effectively. By storing questions in arrays or external files, you can display them sequentially, validate user responses, and calculate a final score. Whether you’re a beginner or an intermediate programmer, this project will strengthen your understanding of text parsing, conditional logic, and error handling.
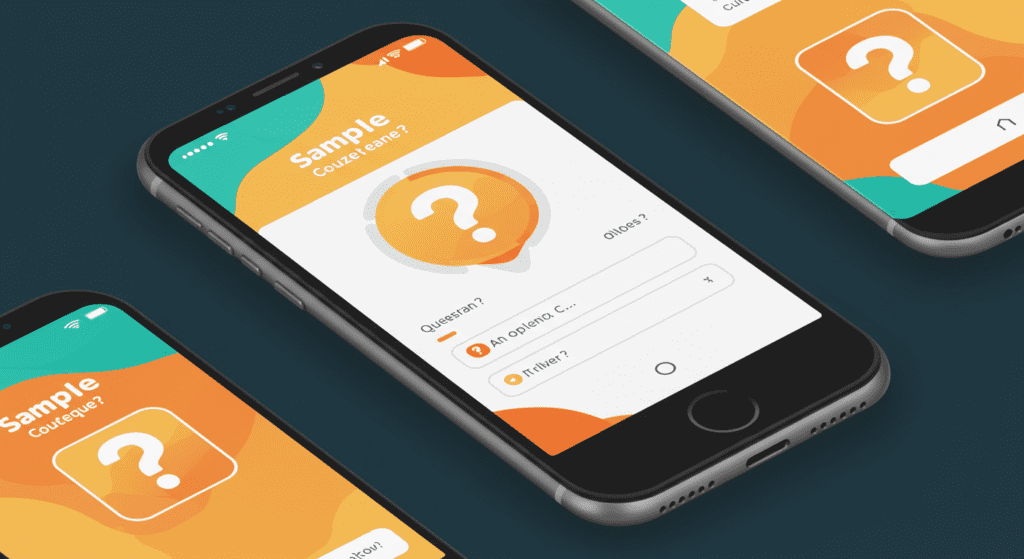
What You Will Learn
This project will help you develop the following skills:
- Question Management: Store prompts and answers using arrays or external files.
- Score Tracking: Maintain and update a cumulative score based on user responses.
- Conditional Logic: Compare user input with correct answers to determine accuracy.
- File Handling (Optional): Load and save questions or user progress for advanced functionality.
- Result Display: Show final scores and provide performance feedback to the user.
Tools Needed to Execute the Project
Tool | Why Is It Needed? |
---|---|
C Compiler | Translates your source code into an executable program to check quiz responses. |
Text Editor or IDE | Helps you organize questions, write code logic, and debug errors efficiently. |
Skills Required for Project Execution
To successfully build this quiz game, you’ll need:
- Basic knowledge of string manipulation.
- Proficiency in loops and conditionals.
- Familiarity with arrays (or basic file I/O if storing questions externally).
- A debugging and test-driven mindset to ensure smooth functionality.
Step-by-Step Guide to Building the Quiz Game
- Create a Question Bank:
Store your questions and correct answers in an array or an external file. For example:cCopychar *questions[] = {“What is 2+2?”, “Is the sky blue?”}; char *answers[] = {“4”, “true”}; - Display Questions and Capture User Input:
Use a loop to display each question and capture the user’s response. - Validate Answers:
Compare the user’s input with the correct answer and update the score accordingly. - Display Results:
At the end of the quiz, show the user’s total score and provide feedback on their performance. - Add Advanced Features (Optional):
- Shuffle questions for variety.
- Implement a timer for added challenge.
- Save user progress to a file for future sessions.
Real-World Applications of the Project
Application | Description |
---|---|
Classroom Quizzes | Teachers and students can use this for interactive tests and revision exercises. |
Interview Preparation | Simulates question-based exercises for job interviews or certification exams. |
Trivia and Learning Apps | Serves as a foundation for more advanced quiz systems that track user progress. |
Conclusion
Building a Quiz Game in C is a rewarding project that enhances your programming skills while creating a practical and interactive application. By managing questions, tracking scores, and validating user input, you’ll gain hands-on experience with essential programming concepts. Whether you’re building this for fun or as a learning tool, the skills you develop will be invaluable for future projects.
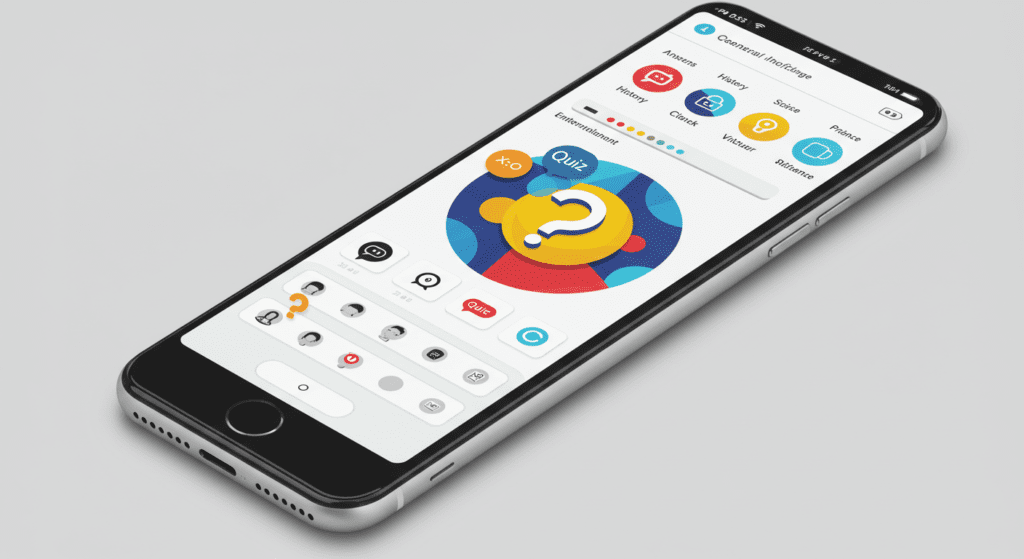
FAQs
1. Can I store questions in an external file?
Yes, you can use file handling in C to load questions and answers from a text file.
2. How can I make the quiz more challenging?
You can add a timer, shuffle questions, or include different types of questions like multiple-choice and true/false.
3. What if the user enters an invalid input?
You can implement error handling to prompt the user to enter a valid response.
4. Can I save user progress?
Yes, by using file handling, you can save user scores and progress for future sessions.