Introduction
Have you ever wondered how calculators work? Creating a simple calculator at home can be a fun and educational DIY project for beginners and tech enthusiasts alike. In this tutorial, we’ll guide you through building a basic calculator using simple materials and programming. Perfect for students, hobbyists, or anyone curious about technology, this project will also give you insights into programming logic and electronics.
By following this step-by-step guide, you’ll learn the basics of creating a calculator, which performs addition, subtraction, multiplication, and division. Let’s dive in!
Table of Contents
- Materials Needed
- How a Simple Calculator Works
- Setting Up Your Workspace
- Step-by-Step Assembly
- Hardware Setup
- Writing the Code
- Testing Your Calculator
- Troubleshooting Tips
- Enhancing Your Calculator
1. Materials Needed
To get started, gather the following materials:
- Microcontroller: Arduino Uno or Raspberry Pi
- Breadboard
- Keypad: 4×4 or 4×3 matrix keypad
- LCD Display: 16×2 LCD screen
- Jumper Wires
- Resistors: 10kΩ for pull-up/pull-down connections
- Power Source: USB cable or battery pack
- Computer: For coding and uploading the program
- Arduino IDE (if using Arduino)
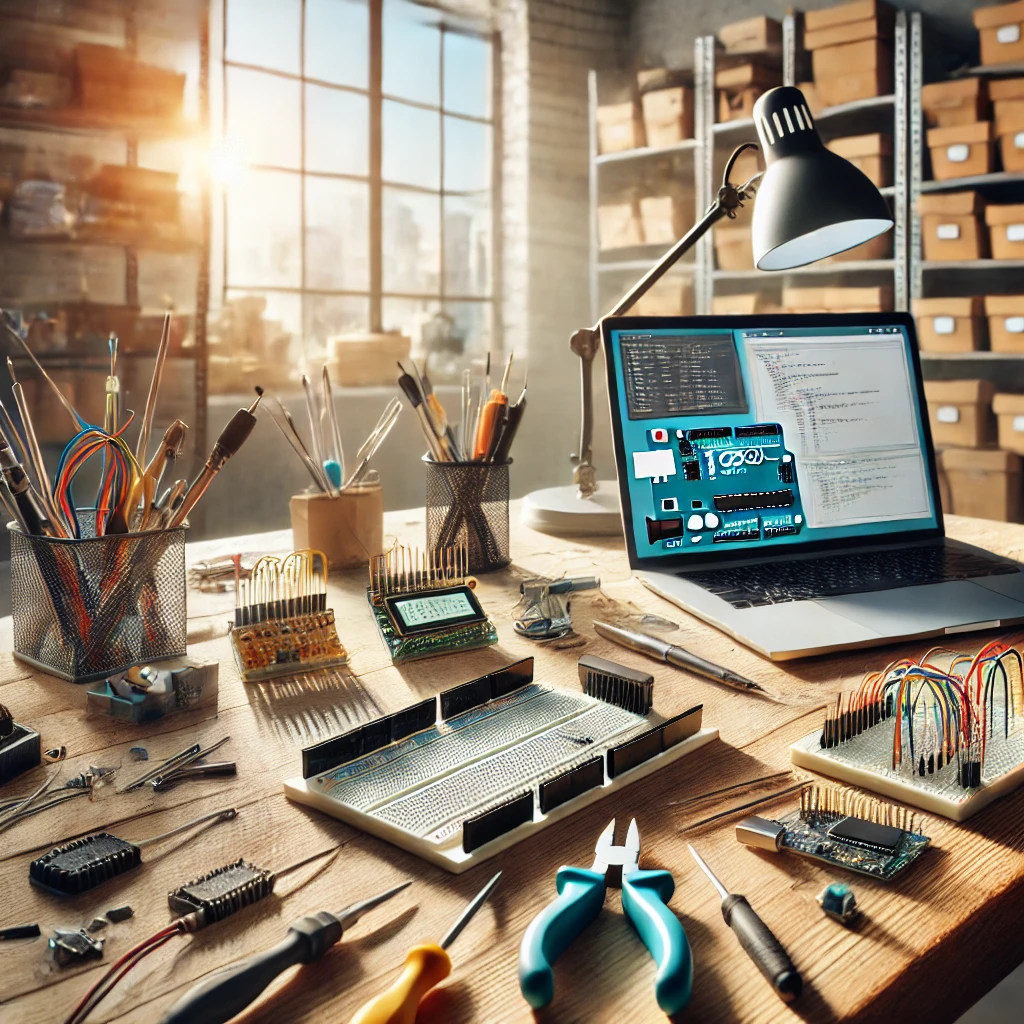
2. How a Simple Calculator Works
A basic calculator takes user input (numbers and operations) and processes it through a program or circuit to display the result. Here’s a simplified flow:
- User presses buttons for numbers and operations.
- The microcontroller interprets the input.
- The program calculates the result.
- The result is displayed on the screen.
3. Setting Up Your Workspace
Ensure you have a clean and organized workspace. Use a non-conductive surface to avoid short circuits and have all materials within reach.
4. Step-by-Step Assembly
Hardware Setup
- Connect the Keypad to the Breadboard:
- Attach the keypad to your breadboard.
- Connect its pins to the microcontroller using jumper wires.
- Connect the LCD Display:
- Wire the LCD to the microcontroller, ensuring proper alignment of data pins.
- Use a potentiometer to adjust the contrast of the LCD display.
- Power the Microcontroller:
- Connect your microcontroller to your computer or a power source.
Writing the Code
- Open your Arduino IDE or Raspberry Pi IDE.
- Write or upload a calculator program. Below is an example for Arduino:
#include <Keypad.h>
#include <LiquidCrystal.h>
const byte ROWS = 4;
const byte COLS = 4;
char keys[ROWS][COLS] = {
{'1','2','3','+'},
{'4','5','6','-'},
{'7','8','9','*'},
{'C','0','=','/'}
};
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2};
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() {
lcd.begin(16, 2);
lcd.print("Simple Calculator");
}
void loop() {
char key = keypad.getKey();
if (key) {
lcd.setCursor(0, 1);
lcd.print(key);
// Add logic for calculations here
}
}
- Upload the code to your microcontroller.
5. Testing Your Calculator
- Power on your microcontroller.
- Press buttons on the keypad to ensure inputs are detected.
- Perform simple calculations to verify accuracy.
6. Troubleshooting Tips
- LCD Not Displaying: Check power connections and contrast settings.
- Keypad Not Responding: Ensure proper wiring and inspect for loose connections.
- Calculation Errors: Debug your code to find and fix logical issues.
7. Enhancing Your Calculator
Take your project to the next level:
- Add support for more complex operations (e.g., square roots, exponents).
- Build a case for your calculator using a 3D printer or cardboard.
- Replace the LCD with an OLED screen for better visuals.
- Implement memory functions to store previous results.
Conclusion
Creating a simple calculator is an excellent way to learn about programming, circuits, and logical thinking. This DIY project not only teaches you valuable skills but also provides a useful tool. Share your creation with others and keep exploring new ways to enhance your project!
Ready to start your calculator project? Gather your materials, follow this guide, and bring your DIY calculator to life!